Каталог с товарами
Создаем файлы моделей models/catalogs.model.php и models/products.model.php
//Файл models/catalogs.model.php class Catalogs extends Model { protected $name = "Разделы каталога"; public $parents = [); protected $model_elements = [ ["Активировать", "bool", "active", ["on_create" => true]), ["Название", "char", "name", ["required" => true]), ["Ссылка", "url", "url", ["unique" => true, "translit_from" => "name"]), ["Родительский раздел", "parent", "parent", ["parent_for" => "Products"]), ["Изображение", "image", "image"], ["Позиция", "order", "order"], ["Описание", "text", "content", ["rich_text" => true]) ); } //Файл models/products.model.php class Products extends Model { protected $name = "Товары каталога"; protected $model_elements = [ ["Активировать", "bool", "active", ["on_create" => true]), ["Название", "char", "name", ["required" => true]), ["Раздел каталога", "enum", "catalog", ["foreign_key" => "Catalogs", "required" => true, "is_parent" => true, "show_parent" => true]), ["Ссылка", "url", "url", ["unique" => true, "translit_from" => "name"]), ["Цена", "int", "price", ["required" => true, "positive" => true]), ["Позиция", "order", "order"], ["Изображения", "multi_images", "images"], ["Рекомендуемые товары", "group", "additional", ["long_list" => true]), ["Описание", "text", "content", ["rich_text" => true]) ); protected $model_display_params = [ "fields_groups" => [ "Основные параметры" => ["active", "name", "catalog", "url", "price", "order", "additional", "images"], "Описание" => ["content"] )); }
Вносим названия моделей в файл config/models.php
$mvActiveModels = ['pages', 'blocks', ... , 'catalogs', 'products'];
Создаем SQL таблицы в базе данных, после чего модели смогут работать в административной панели
CREATE TABLE `catalogs` ( `id` int(11) NOT NULL, `active` tinyint(1) NOT NULL, `name` varchar(200) NOT NULL, `url` varchar(200) NOT NULL, `order` int(11) NOT NULL, `parent` int(11) NOT NULL, `content` text NOT NULL, `image` varchar(250) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=utf8; ALTER TABLE `catalogs` ADD PRIMARY KEY (`id`), ADD KEY `parent_active` (`parent`,`active`), ADD KEY `url_active` (`url`,`active`), ADD KEY `parent` (`parent`); ALTER TABLE `catalogs` MODIFY `id` int(11) NOT NULL AUTO_INCREMENT; CREATE TABLE `products` ( `id` int(11) NOT NULL, `name` varchar(250) NOT NULL, `active` tinyint(1) NOT NULL, `order` int(11) NOT NULL, `catalog` int(11) NOT NULL, `url` varchar(200) NOT NULL, `price` int(11) NOT NULL, `content` text NOT NULL, `images` text NOT NULL, `additional` varchar(250) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=utf8; ALTER TABLE `products` ADD PRIMARY KEY (`id`), ADD UNIQUE KEY `id_active` (`id`,`active`), ADD KEY `url_active` (`url`,`active`), ADD KEY `catalog_active` (`catalog`,`active`); ALTER TABLE `products` MODIFY `id` int(11) NOT NULL AUTO_INCREMENT;
Создаем файлы шаблонов views/catalog/view-catalog.php и views/catalog/view-product.php, а также вносим маршрут к шаблонам в файл config/routes.php
$mvFrontendRoutes = [ ... , "catalog/*/" => "catalog/view-catalog.php", "product/*/" => "catalog/view-product.php" ];
Добавляем методы в файл модели Catalogs
public function defineCatalogPage(Router $router) { $url_parts = $router -> getUrlParts(); $record = false; if(count($url_parts) == 2 && $url_parts[0] == "catalog") if(is_numeric($url_parts[1])) $record = $this -> find(["id" => $url_parts[1], "active" => 1]); else $record = $this -> find(["url" => $url_parts[1], "active" => 1]); if($record) { $this -> id = $record -> id; $this -> parents = array_keys($this -> getParents($record -> id)); } return $record; } public function displayCatalogTreeMenu($parent) { $rows = $this -> select(["parent" => $parent, "active" => 1, "order->asc" => "order"]); $html = ""; foreach($rows as $row) { $children = 0; $css_class = ""; $open = in_[$row['id'], $this -> parents]; $children = $this -> countRecords(["parent" => $row['id'], "active" => 1]); $css_class = $children ? "has-children" : ""; $css_class .= ($row['id'] == $this -> id) ? " active" : ""; $css_class .= ($children && in_[$row['id'], $this -> parents]) ? " open" : ""; $css_class = $css_class ? ' class="'.trim($css_class).'"' : ""; $url = $this -> root_path."catalog/".($row['url'] ? $row['url'] : $row['id'])."/"; $html .= "
- \n".$this -> displayCatalogTreeMenu($row['id'])."
\n";
$html .= "\n";
$html .= $this -> cropImage($this -> getFirstImage($row['image']), 230, 200)."\n";
$html .= "".$row['name']."";
$html .= "
\n";
}
return $html;
}
Добавляем методы в файл модели Products
public function defineProductPage(Router $router) { $url_parts = $router -> getUrlParts(); $record = false; if(count($url_parts) == 2) if(is_numeric($url_parts[1])) $record = $this -> find(["id" => $url_parts[1], "active" => 1]); else $record = $this -> find(["url" => $url_parts[1], "active" => 1]); return $record; } public function display($params) { $params["active"] = 1; if(isset($this -> pager)) $params["limit->"] = $this -> pager -> getParamsForSelect(); $rows = $this -> select($params); $html = ""; foreach($rows as $row) { $url = $this -> root_path."product/".($row["url"] ? $row["url"] : $row["id"])."/"; $image = $this -> resizeImage($this -> getFirstImage($row["images"]), 250, 250); $html .= "\n"; $html .= "\n"; $html .= "\n"; $html .= ""; } return $html; } public function displayAdditionalProducts($product) { if($product -> additional) $params = ["id->in" => $product -> additional, "limit->" => 4]; else $params = ["id!=" => $product -> id, "catalog" => $product -> catalog, "limit->" => 4]; $count = $this -> countRecords($params); if($count) { $html = "".$row["price"]."\n"; $html .= "Рекомендуем посмотреть\n"; $html .= "\n"; $html .= $this -> display($params); $html .= "\n"; return $html; } }
Содержимое файла view-catalog.php
$catalog = $mv -> catalogs -> defineCatalogPage($mv -> router); $mv -> display404($catalog); $count_products = $mv -> products -> countRecords(["catalog" => $catalog -> id, "active" => 1]); //Если в каталоге есть товары, то включаем постраничную разбивку if($count_products) { $current_page = $mv -> router -> defineCurrentPage("page"); $mv -> products -> runPager($count_products, 20, $current_page); $path = $mv -> root_path."catalog/".($catalog -> url ? $catalog -> url : $catalog -> id)."/"; } $mv -> seo -> mergeParams($catalog, "name"); include $mv -> views_path."main-header.php";include $mv -> views_path."main-footer.php";echo $catalog -> name;
if(!$count_products) //Вывод подразделов { echo "\n"; echo $mv -> catalogs -> display($catalog -> id)."\n"; } else //Вывод товаров если они есть { echo "\n"; echo $mv -> products -> display(["catalog" => $catalog -> id])."\n"; if($count_products && $mv -> products -> pager -> hasPages()) { echo "\nСтраницы: \n"; echo $mv -> products -> pager -> display($path, false); echo "\n"; } }
Содержимое файла view-product.php
$product = $mv -> products -> defineProductPage($mv -> router); $mv -> display404($product); $mv -> seo -> mergeParams($product, "name"); include $mv -> views_path."main-header.php";include $mv -> views_path."main-footer.php";$image = $mv -> products -> getFirstImage($product -> images); echo $mv -> products -> resizeImage($image, 450, 450, ["title" => $product -> name]);echo $product -> name;
echo $product -> price;echo $product -> content;echo $mv -> products -> displayAdditionalProducts($product);
Предыдущий раздел
Вопросы и ответы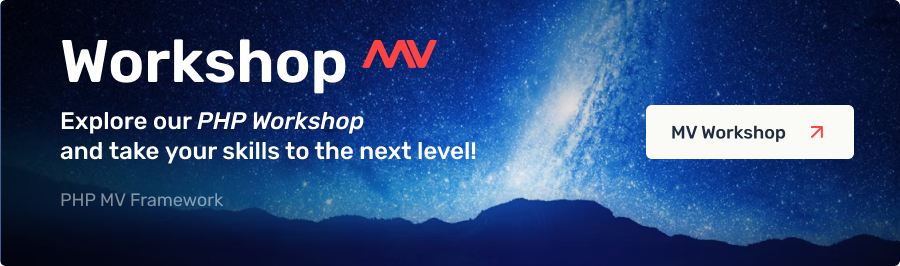